15 interesting Python modules to learn in 2016
15 interesting Python modules to learn in 2016 |
In this article I will give you an introduction into some Python modules I think of as useful. Naturally this can vary in your case but anyway it is a good idea to look at them, maybe you will use them in the future.
I won't give a thorough introduction for each module because it would blow-up the boundaries of this article. However I will try to give you a fair summary on the module: what id does and how you can use it in the future if you are interested. For a detailed introduction you have to wait for either future articles which explain these libraries in more depth or you have to search the web to find resources. And there are plenty of good resources out there.
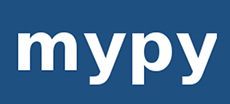
In some languages static typing is the only way to go. In Python there is dynamic typing which means once a variable has assigned a value of a given type (int for example) it can later become a value which is from another type (string). However in some applications this leads to errors because code blocks expect values from a given type and you pass in something else instead.
Python 3.5 introduced the standard for type annotations where you can annotate your code to display what type the function parameters should have and what type the function returns for example. However type checking is only an annotation which you see in the code but is removed when this code gets executed.
>>> def add(a: int, b: int) -> int:
... return a b
...
The example function above adds two variables. The type hint shows that the expected numbers are from type int and the result is an int too.
To demonstrate that the type hints are only for the developers let's call this function:
>>> add(1, 5)
6
>>> add('a', '3')
'a3'
There is no problem if you provide other types to the function as long as Python can use the operator on both variables.
Now mypy is a library which looks for such type hints and gives you warnings if you try to call a function with the wrong type. Even if your static type checking with mypy fails you can run your application with your interpreter of choice.
Let's convert the example above to a Python module and call it typing_test.py:
__author__ = 'GHajba'
def add(a: int, b: int) -> int:
return a b
if __name__ == '__main__':
print(add(1, 5))
print(add('a', '3'))
As you can see, I call the function with the same parameters as previously and I expect that I get some type warnings:
mypy typing_test.py
typing_test.py:6: error: Argument 1 to "add" has incompatible type "str"; expected "int"
typing_test.py:6: error: Argument 2 to "add" has incompatible type "str"; expected "int"
And yes, there it is. If I run the script (as mentioned above) it executes:
python3 typing_test.py
6
a3
6
a3
An interesting (and in my eyes not a very good) result comes when you execute type checking on a file which has no type errors: you get no output. I would expect at least one line telling me that 0 errors were found. To satisfy my needs I have to run mypy with the -v flag for verbose mode however this gives too much information and if you get some errors the screen is full with data you are not interested in. I hope this will change in a future version, I am currently using 0.4.3.
You can find a quick documentation about mypy athttps://github.com/python/mypy(including how to install this library).
Nose (https://reahttps://readthedocs.org/) is a testing library. As their homepage states: "nose is nicer testing for python". And their tagline is: "nose extends unit test to make testing easier."
And I know you do not want to write unit tests. You are a Python developer who is a rebel and does not want to compile with Java-like developers who have to write tests to get their code accepted.
Anyway I do not want to go into detail that you must or don't have to write tests (sometimes I even don't test my Java code) but sometimes it is a good approach to write tests -- the best use case for this is when you write a complex block of code. So let's install nose:
pip install nose
For an example let's create a module to test and call it overcomplicated.py:
author__ = 'GHajba'
def multiply(a, b):
if a == 0:
return 0
return a multiply(a-1, b)
As the name mentions this module contains overcomplicated things and, well, the contents are really over-complicated. Now we want to test this module. For this let's create a simple test file and call it overcomplicated_test.py (and I know there are errors in the assertion but they are there for good):
from overcomplicated import multiply
__author__ = 'GHajba'
def test_simple():
assert multiply(3, 4) == 12
def test_with_0():
assert multiply(0, 0) == 1
assert multiply(0, 12) == 0
assert multiply(45, 0) == 0
assert multiply(-3, 0) == 0
def test_negative():
assert multiply(-1, 3) == -3
assert multiply(-9, -2) == 18
assert multiply(42, -3) == -125
Well, the test itself is not as complicated than the module to test but this is good for a simple introduction. We test here some corner cases like multiplying with 0, multiplying 0 and multiplication with negative numbers.
Now let's see how we can utilizenose and why it is useful for testing:
nosetest overcomplicated_test.py
If you run the test above you will get an error and one failed test. And these tests test negative numbers. Why is this?
File "/Users/GHajba/dev/python/overcomplicated.py", line 4, in multiply
return b multiply(a-1, b)
File "/Users/GHajba/dev/python/overcomplicated.py", line 4, in multiply
return b multiply(a-1, b)
File "/Users/GHajba/dev/python/overcomplicated.py", line 2, in multiply
if a == 0:
RecursionError: maximum recursion depth exceeded in comparison
----------------------------------------------------------------------
Ran 3 tests in 0.018s
FAILED (errors=2)
That's because of the stop-condition in the multiply function: the recursion stops if a has the value of 0. However with negative multiplier there is a problem.
Now let's correct the script and re-run the tests:
.FF
======================================================================
FAIL: overcomplicated_test.test_with_0
----------------------------------------------------------------------
Traceback (most recent call last):
File "/Library/Frameworks/Python.framework/Versions/3.5/lib/python3.5/site-packages/nose/case.py", line 198, in runTest
self.test(*self.arg)
File "/User/GHajba/dev/python/overcomplicated_test.py", line 7, in test_with_0
assert multiply(0,0) == 1
AssertionError
======================================================================
FAIL: overcomplicated_test.test_negative
----------------------------------------------------------------------
Traceback (most recent call last):
File "/Library/Frameworks/Python.framework/Versions/3.5/lib/python3.5/site-packages/nose/case.py", line 198, in runTest
self.test(*self.arg)
File "/Users/GHajba/dev/python/overcomplicated_test.py", line 15, in test_negative
assert multiply(42, -3) == -125
AssertionError
----------------------------------------------------------------------
Ran 3 tests in 0.001s
FAILED (failures=2)
The 2 failures are there however now it is not a problem with recursion. As you can see, the test is failing but it shows you the spots where the errors happened. Now let's fix all the errors in the tests and run the script again:
nosetests -v overcomplicated_test.py
overcomplicated_test.test_simple ... ok
overcomplicated_test.test_with_0 ... ok
overcomplicated_test.test_negative ... ok
----------------------------------------------------------------------
Ran 3 tests in 0.001s
OK
Now we are done and all tests are finished without errors. The -v flag tells nose to display a verbose result on the tests which were executed.
This is a module which comes with your Python installation. This library arrived in this article because it is useful if you just want to start a simple HTTP server where you can try your homepage and see if the internal links are working.
To start a server just execute the following command from your command line:
python -m http.server
Note that this module is only available in Python 3. Well, this sentence was not fully true, the server is available for Python 2 too but it has to be used in a bit different way:
python -m SimpleHTTPServer
The default port both versions listen to is 8000. If you want to use a different port (like 8080) you can put the port number at the end of the starting command.
For Python 3 it looks like this:
python -m http.server 8080
And for Python 2 like this:
python -m SimpleHTTPServer 8080
If the server is running, you can see all the requests logged to the console (if you do not redirect the output into a file of course). This gives you an opportunity to see if your template you downloaded for free does not contain a malicious part where you are redirected or getting unwanted content.
Click the link bellow to read more .... CLICK
Leave a Comment